As part of DrupalEasy's Professional Module Development (PMD) course, I teach students how to configure an efficient development environment using either DDEV or Lando combined with either PhpStorm or Visual Studio Code. We go rather deep into this topic, often devoting 4-5 hours of class time in getting everyone set up.
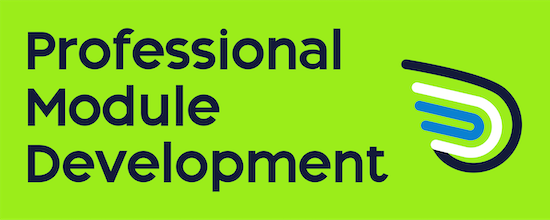
As the material is part of our proprietary curriculum for which our clients/students pay tuition , I've been reluctant to share it directly: However, over the past couple of years, the DDEV + Visual Studio Code combination has been more and more common in Drupal development and I've been persuaded to share a summary of my recommended setup.
(I have also, for quite some time, been providing a summary of this material in various free Drupal camp workshops as well as Drupal camp presentations.)
The goal of this blog post is to guide you through maximizing your Drupal development environment by integrating Visual Studio Code with DDEV by connecting it directly to the DDEV web container's filesystem.
While this is not the most common method of using Visual Studio Code with DDEV, I have found (and many of our PMD students agree) that this provides the easiest access to full IDE integration with tools like PhpStan and phpcs (among others.)
Much of the content in this document is also valid for other Docker-based local development environments (including Lando, Docksal, custom Docker). The main differences are related to directory paths.
Prerequisites
Before you can fully leverage the information in this document, be sure that:
- You are comfortable using DDEV on a daily basis.
- Your Drupal project includes the core development dependencies (via https://packagist.org/packages/drupal/core-dev).
- Your project is configured to run phpcs, phpcbf, phpstan, and phpunit from the command line within the DDEV web container, including all relevant configuration files.
- Finally, it is strongly recommended to use a settings.local.php file for your local development environment (here's why!)
Unleashing the power of remote development
A key aspect of this setup is leveraging Visual Studio Code's Remote Explorer and Dev Containers extensions. The general idea is to connect Visual Studio Code directly to your project's code base within the DDEV web container instead of accessing the files on your host operating system.
The primary advantage of this approach is that it allows other Visual Studio Code extensions to easily access and execute command-line tools that reside within the container. For instance, extensions for code quality like phpcs and PhpStan, which are typically installed within your Drupal project via the drupal/core-dev dependencies, can be directly invoked by Visual Studio Code without requiring complex configurations or path mappings to your host system's executables. This ensures that the code quality checks are performed using the exact tools and versions defined in your project's container.
Essential Visual Studio Code extensions
To achieve this integration as well as provide an efficient Drupal development environment, the following Visual Studio Code extensions are recommended:
- Remote explorer: ms-vscode.remote-explorer
- Dev Containers: ms-vscode-remote.remote-containers
- PHP Debug: xdebug.php-debug
- PHP DocBlocker: neilbrayfield.php-docblocker
- PHP Intelephense: bmewburn.vscode-intelephense-client
- PHP Sniffer & Beautifier: ValeryanM.vscode-phpsab
- phpstan: SanderRonde.phpstan-vscode
- PHPUnit: emallin.phpunit
- Drupal Smart Snippets: andrewdavidblum.drupal-smart-snippets
- Twig Language 2: mblode.twig-language-2
Note that when using this technique, the excellent DDEV Manager extension is not used as it normally operates from the host OS, and not inside the DDEV web container.
Making the connection
The key to making all this work is having Visual Studio Code connect directly to the project files in the DDEV web container and not in the host operating system. To do so, after launching Visual Studio Code, click on the Remote Explorer icon in the sidebar, then select Dev Containers from the select list, then select the web container for your project.
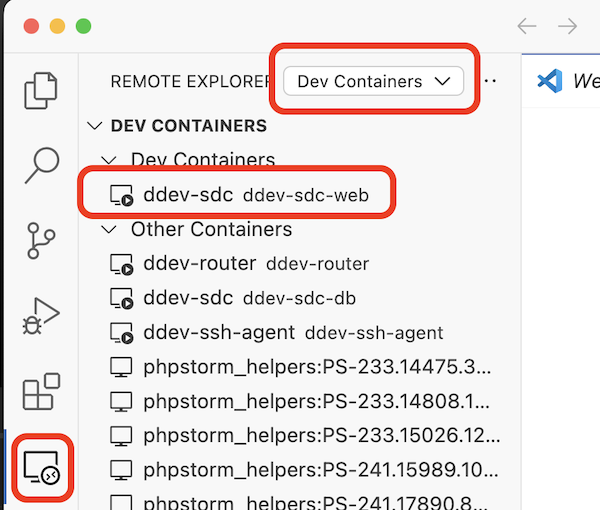
Once connected, click the Extensions icon in the sidebar, and install in the container any of the extensions listed above that require it (not all extensions need to be installed in the container.)
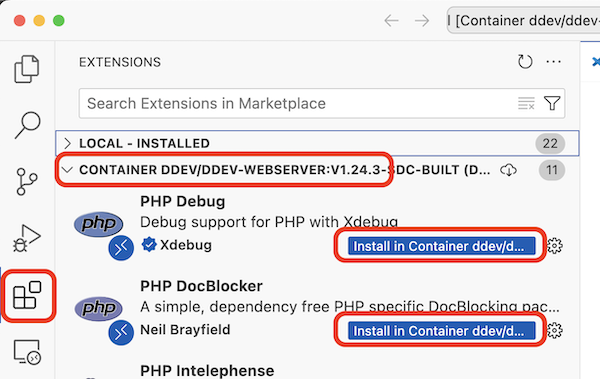
Finally, click the Explorer icon in the sidebar and navigate to the project root - usually /var/www/html.
At this point, while Visual Studio Code is running in your host OS, it only "sees" the DDEV web container. Which means that when you open the Visual Studio Code Terminal, you are executing commands in the DDEV web container by default.
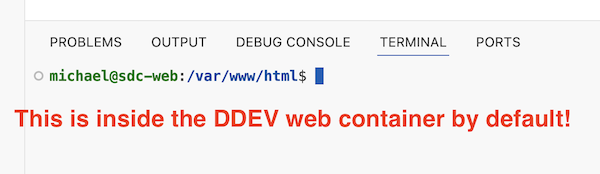
From this command line, Drush, Composer, Git, npm, and other installed binaries can be run directly - without the need to prepend them with ddev or ddev exec (ddev .)
Understanding Visual Studio Code settings
In order to configure everything effectively, it is important to understand that Visual Studio Code supports two types of settings:
- User settings: These are global settings that apply to all your Visual Studio Code projects unless overridden by workspace settings.
- Workspace settings: These settings are specific to a particular project and will override any conflicting user settings. These settings are typically stored in a .vscode/settings.json file within your project's root directory.
Recommended workspace settings
Here is the recommended .vscode/settings.json configuration for DDEV in your project root. Note that if you already have settings in this file, you'll likely want to combine what is provided below with your current settings.
{
/* DDEV web container */
/* PHP Intelephense */
"intelephense.environment.phpVersion": "8.3",
/* PHP Linting */
"php.validate.executablePath": "/usr/bin/php",
/* PHP Sniffer & Beautifier */
"phpsab.executablePathCS": "/var/www/html/vendor/bin/phpcs",
"phpsab.executablePathCBF": "/var/www/html/vendor/bin/phpcbf",
"phpsab.standard": "/var/www/html/phpcs.xml",
"phpsab.snifferEnable": true,
"phpsab.fixerEnable": true,
"phpsab.snifferMode": "onType",
"phpsab.debug": false,
"phpsab.fixerArguments": [],
/* phpstan */
"phpstan.enabled": true,
"phpstan.showProgress": true,
"phpstan.binPath": "/var/www/html/vendor/bin/phpstan",
"phpstan.configFile": "/var/www/html/phpstan.neon"
}
Some notes:
- intelephense.environment.phpVersion: Specifies the PHP version used in the DDEV container, aiding PHP Intelephense with accurate code intelligence.
- php.validate.executablePath: Defines the path to the PHP executable within the DDEV container, used for basic PHP linting.
- phpsab.executablePathCS and phpsab.executablePathCBF: Point to the phpcs and phpcbf executables within the DDEV container's vendor directory, used by the PHP Sniffer & Beautifier extension for code standard checking and fixing.
- phpsab.standard: Specifies the path to your project's PHPCS ruleset file (e.g., phpcs.xml) within the DDEV container.
- phpsab.snifferEnable, phpsab.fixerEnable, phpsab.snifferMode, phpsab.debug, phpsab.fixerArguments: Configuration options for the PHP Sniffer & Beautifier extension, controlling its behavior for real-time code analysis and formatting.
- phpstan.enabled, phpstan.showProgress, phpstan.binPath, phpstan.configFile: Settings for the phpstan extension, specifying whether it's enabled, whether to show progress, and the paths to the phpstan executable and configuration file within the DDEV container.
Recommended user settings
For some of the previously mentioned extensions, there are some user-level settings you might want to merge into your existing Visual Code Studio User settings.
To ensure the PHP Sniffer & Beautifier is the default formatter for PHP files, add the following:
"[php]": {
"editor.defaultFormatter": "valeryanm.vscode-phpsab"
}
To prioritize Drupal Smart Snippets for code completion suggestions, add the following:
"editor.snippetSuggestions": "top"
To ensure PHP Intelephense uses the correct Drupal document root for code intelligence, add the following (this assumes your docroot is web):
"intelephense.environment.documentRoot": "web/index.php"
Summary
By following these recommendations and configuring your Visual Studio Code environment as described above, you can significantly enhance your Drupal development efficiency by taking full advantage of your local development environment's capabilities directly within Visual Studio Code.
Thanks to Randy Fay and Stas Zhuk (DDEV maintainers) for reviewing this blog post prior to publication.
AI was used to create the initial outline for this blog post using DrupalEasy's Professional Module Development curriculum as the source material. We support AI disclosure statements on all content that is posted to Drupal Planet.
Comments
Thanks! Would you recommend…
Thanks! Would you recommend the same approach with Phpstorm, of wiring it up to do everything within the container rather than the host OS? I've used Phpstorm, with it presumably just talking to the host OS, without any issues so far. Though maybe that's because I haven't got as far into using the CLI tools like PhpStan and phpcs. Just wondering if I might be missing out on more too though.
PhpStorm is a bit different …
PhpStorm is a bit different - it has the concept of CLI interpreters that can run commands directly in the Docker container. See https://iamdroid.net/blog/dev-tools#ddev-phpstorm-configuration
Add new comment